In this section, we will see how to implement a carousel slider in Laravel 9 using Tailwind CSS. Throughout this tutorial, we’ll cover both static and dynamic sliders with Laravel 9.
Example 1
Run below command in your terminal to create laravel project.
composer create-project --prefer-dist laravel/laravel laravel-slider
Create Slider Controller
Run below command to create slider controller.
php artisan make:controller SliderController
app/Http/Controllers/SliderController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class SliderController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('sliders.index');
}
}
Create Slider Route
Route::get('sliders', [SliderController::class,'index'])->name('sliders');
Add @stacks in main blade file
resources/views/layouts/app.blade.php
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<!-- Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Nunito:wght@400;600;700&display=swap">
@stack('styles')
<!-- Scripts -->
@vite(['resources/css/app.css', 'resources/js/app.js'])
</head>
<body class="font-sans antialiased">
<div class="min-h-screen bg-gray-100">
@include('layouts.navigation')
<!-- Page Heading -->
<header class="bg-white shadow">
<div class="px-4 py-6 mx-auto max-w-7xl sm:px-6 lg:px-8">
{{ $header }}
</div>
</header>
<!-- Page Content -->
<main>
{{ $slot }}
</main>
</div>
@stack('scripts')
</body>
</html>
Add Swiper slider using @push in blade
views/sliders/index.blade.php
<x-app-layout>
@push('styles')
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css" />
@endpush
<x-slot name="header">
<h2 class="text-xl font-semibold leading-tight text-gray-800">
{{ __('Laravel 9 Slider') }}
</h2>
</x-slot>
<div class="swiper mySwiper">
<div class="swiper-wrapper">
<div class="swiper-slide">
<img class="object-cover w-full h-96" src="https://source.unsplash.com/user/erondu/3000x900" alt="image" />
</div>
<div class="swiper-slide">
<img class="object-cover w-full h-96" src="https://source.unsplash.com/collection/190727/3000x900" alt="image" />
</div>
<div class="swiper-slide">
<img class="object-cover w-full h-96" src="https://source.unsplash.com/collection/190728/3000x900" alt="image" />
</div>
</div>
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
<div class="swiper-pagination"></div>
</div>
@push('scripts')
<!-- Swiper JS -->
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper(".mySwiper", {
cssMode: true,
navigation: {
nextEl: ".swiper-button-next",
prevEl: ".swiper-button-prev",
},
pagination: {
el: ".swiper-pagination",
},
mousewheel: true,
keyboard: true,
});
</script>
@endpush
</x-app-layout>
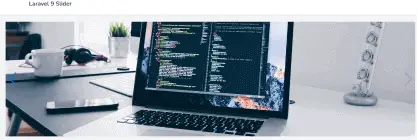
Run laravel and vite server
php artisan serve
// and
npm run dev
Example 2
Add Dynamic slider in Laravel 9 with Tailwind CSS
Create Slider Model Controller and Routes.
php artisan make:model Slider -mcr
create_sliders_table.php
public function up()
{
Schema::create('sliders', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('image');
$table->timestamps();
});
}
app/Models/Slider.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Slider extends Model
{
use HasFactory;
protected $fillable = [
'name',
'image'
];
}
Store Dynamic image Slider
app/Http/Controllers/SliderController.php
<?php
namespace App\Http\Controllers;
use App\Models\Slider;
use Illuminate\Http\Request;
class SliderController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$sliders = Slider::all();
return view('sliders.index', compact('sliders'));
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('sliders.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'name' => 'required|string|max:255',
'image' => 'required|image|mimes:jpg,png,jpeg,gif,svg|max:2048'
]);
if ($request->hasFile('image')) {
$image_path = $request->file('image')->store('image', 'public');
}
Slider::create([
'name' => $request->name,
'image' => $image_path
]);
return redirect()->route('sliders.index');
}
/**
* Display the specified resource.
*
* @param \App\Models\Slider $slider
* @return \Illuminate\Http\Response
*/
public function show(Slider $slider)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Models\Slider $slider
* @return \Illuminate\Http\Response
*/
public function edit(Slider $slider)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Slider $slider
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Slider $slider)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Slider $slider
* @return \Illuminate\Http\Response
*/
public function destroy(Slider $slider)
{
//
}
}
Create Dynamic slider
sliders/create.blade.php
<x-app-layout>
<x-slot name="header">
<h2 class="text-xl font-semibold leading-tight text-gray-800">
{{ __('SLider Create') }}
</h2>
</x-slot>
<div class="py-12">
<div class="mx-auto max-w-7xl sm:px-6 lg:px-8">
<div class="overflow-hidden bg-white shadow-sm sm:rounded-lg">
<div class="p-6 bg-white border-b border-gray-200">
<form method="POST" action="{{ route('sliders.store') }}" enctype="multipart/form-data">
@csrf
<div class="mb-6">
<label class="block">
<span class="text-gray-700">Name</span>
<input type="text" name="name"
class="block w-full @error('name') border-red-500 @enderror mt-1 rounded-md"
placeholder="" value="{{old('name')}}" />
</label>
@error('title')
<div class="text-sm text-red-600">{{ $message }}</div>
@enderror
</div>
<div class="mb-4">
<label class="block">
<span class="text-gray-700">Image</span>
<input type="file" name="image"
class="block w-full mt-1 rounded-md"
placeholder="" />
</label>
@error('image')
<div class="text-sm text-red-600">{{ $message }}</div>
@enderror
</div>
<button type="submit"
class="text-white bg-blue-600 rounded text-sm px-5 py-2.5">Submit</button>
</form>
</div>
</div>
</div>
</div>
</x-app-layout>
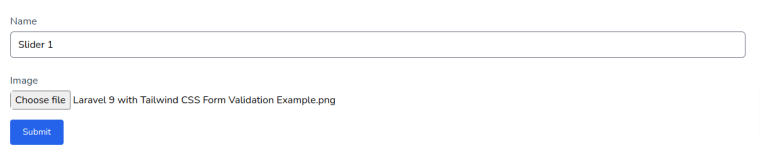
Add Dynamic slider in index file
sliders/index.blade.php
<x-app-layout>
@push('styles')
<link
rel="stylesheet"
href="https://unpkg.com/swiper/swiper-bundle.min.css"
/>
@endpush
<x-slot name="header">
<h2 class="text-xl font-semibold leading-tight text-gray-800">
{{ __('Laravel 9 Slider') }}
</h2>
</x-slot>
<div class="mt-6 mx-60 swiper mySwiper">
<div class="swiper-wrapper">
@foreach ($sliders as $slider)
<div class="swiper-slide">
<img
class="object-cover w-full h-full"
src="{{ asset('/storage/' . $slider->image) }}"
alt="{{ $slider->title }}"
/>
</div>
@endforeach
</div>
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
<div class="swiper-pagination"></div>
</div>
@push('scripts')
<!-- Swiper JS -->
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper(".mySwiper", {
cssMode: true,
navigation: {
nextEl: ".swiper-button-next",
prevEl: ".swiper-button-prev",
},
pagination: {
el: ".swiper-pagination",
},
mousewheel: true,
keyboard: true,
});
</script>
@endpush
</x-app-layout>
Run the laravel and vite server
php artisan serve
// and
npm run dev
[…] How to Use Carousel Slider in Laravel 9 Example […]