In this section, we will connect a Laravel API with Nuxt.js 3. We will fetch data from the Laravel API in Nuxt 3 using useFetch
and useAsyncData
.
Setup Laravel Api
Create laravel project and connect with database.
composer create-project laravel/laravel laravel-api
Create Fake Data and Migrate Seeder
DatabaseSeeder.php
<?php
namespace Database\Seeders;
use Illuminate\Database\Console\Seeds\WithoutModelEvents;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
\App\Models\User::factory(10)->create();
}
}
Migrate the database with seeder.
php artisan migrate:fresh --seed
Create Api Controller and Api Route
Run below command to create user Api controller.
php artisan make:controller Api/UserController
app/Http/Controllers/Api/UserController.php
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index()
{
$users = User::all();
// return $users;
return response()->json($users);
}
}
Create users api route.
routes/api.php
<?php
use App\Http\Controllers\Api\UserController;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware(['auth:sanctum'])->get('/user', function (Request $request) {
return $request->user();
});
Route::get('/users',[UserController::class,'index']);
Run Laravel server and leave it.
php artisan serve
Nuxt 3 Data Fetching Using Laravel Api
Create new nuxt 3 app.
npx nuxi init frontend
There are two ways to fetch API data in Nuxt.js 3: you can use useAsyncData
or useFetch
.
- Fetch Laravel API data using
useAsyncData
in Nuxt 3.
Remove <NuxtWelcome />
in the app.vue
file.
<template>
<div>
<h1>Laravel Api with Nuxt 3 Fetch Api Data</h1>
<ul v-for="user in users" :key="user.id">
<li>Id: {{ user.id }}</li>
<li>Name: {{ user.name }}</li>
<li>Email: {{ user.email }}</li>
</ul>
</div>
</template>
<script setup>
const { data: users } = await useAsyncData('users', () =>
$fetch(`http://localhost:8000/api/users`)
);
</script>
Fetch Laravel API data using useFetch
in Nuxt 3.
<template>
<div>
<h1>Laravel Api with Nuxt 3 Fetch Api Data</h1>
<ul v-for="user in users" :key="user.id">
<li>Id: {{ user.id }}</li>
<li>Name: {{ user.name }}</li>
<li>Email: {{ user.email }}</li>
</ul>
</div>
</template>
<script setup>
const { data: users } = await useFetch(`http://localhost:8000/api/users`);
</script>
http://localhost:3000/
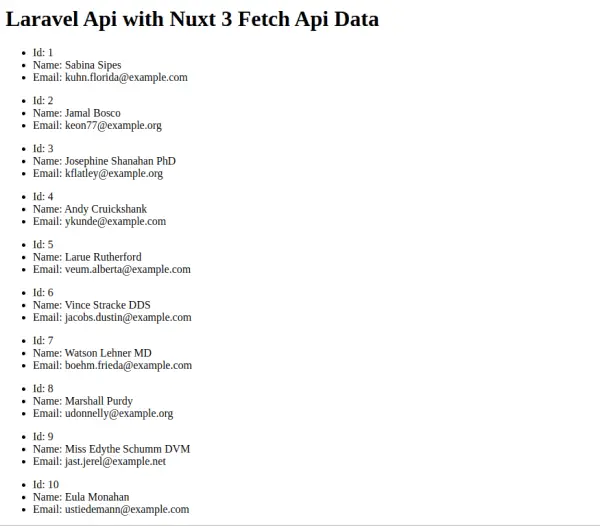
If the data is not showing, restart the Nuxt 3 server, and then in the terminal, run the Laravel app again.
npm run dev