In this section, we will learn how to use a toggle switch in Laravel 10 with FilamentPHP v3. A toggle switch is useful for activating and deactivating fields, such as admin draft posts.
You can use a toggle switch via the Toggle component.
use Filament\Forms\Components\Toggle;
Toggle::make('is_active')
If you’re saving a boolean value using Eloquent, make sure to add a boolean cast to the model property.
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $casts = [
'is_active' => 'boolean',
];
// ...
}
You can also use toggle column via ToggleColumn component.
use Filament\Tables\Columns\ToggleColumn;
ToggleColumn::make('is_active')
Additionally, we will demonstrate how to add a toggle switch button in a Laravel 10 Filament v3 CRUD app.
Laravel 10 Filament v3 CRUD Operation Example
Filament/Resources/BlogResource.php
<?php
namespace App\Filament\Resources;
use App\Filament\Resources\BlogResource\Pages;
use App\Filament\Resources\BlogResource\RelationManagers;
use App\Models\Blog;
use Filament\Forms;
use Filament\Forms\Form;
use Filament\Resources\Resource;
use Filament\Tables;
use Filament\Tables\Table;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Components\Textarea;
use Filament\Tables\Columns\TextColumn;
use Filament\Forms\Components\Section;
use Filament\Forms\Components\RichEditor;
use Filament\Tables\Columns\ToggleColumn;
use Filament\Forms\Components\Toggle;
use Filament\Tables\Columns\ImageColumn;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
class BlogResource extends Resource
{
protected static ?string $model = Blog::class;
protected static ?string $navigationIcon = 'heroicon-o-rectangle-stack';
public static function form(Form $form): Form
{
return $form
->schema([
Section::make()
->schema([
TextInput::make('title')->required(),
RichEditor::make('content')->required(),
Toggle::make('is_active')
])
]);
}
public static function table(Table $table): Table
{
return $table
->columns([
TextColumn::make('id')->sortable(),
TextColumn::make('title')->searchable()->sortable(),
TextColumn::make('created_at')->searchable()->sortable(),
ToggleColumn::make('is_active')->searchable(),
TextColumn::make('content')->limit(20)->markdown()->sortable()->searchable(),
])
->filters([
//
])
->actions([
Tables\Actions\EditAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
]),
])
->emptyStateActions([
Tables\Actions\CreateAction::make(),
]);
}
public static function getRelations(): array
{
return [
//
];
}
public static function getPages(): array
{
return [
'index' => Pages\ListBlogs::route('/'),
'create' => Pages\CreateBlog::route('/create'),
'edit' => Pages\EditBlog::route('/{record}/edit'),
];
}
}
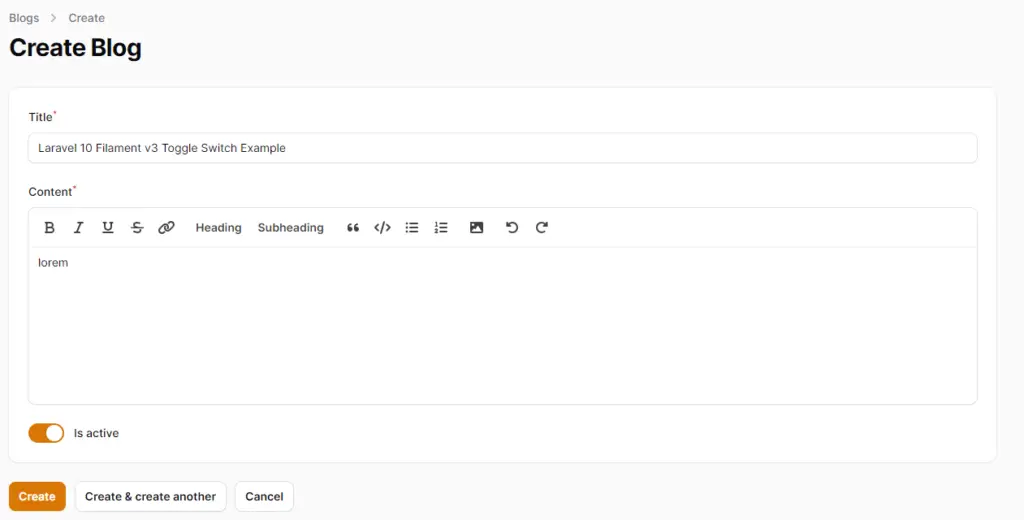
Toggle switch button in table.
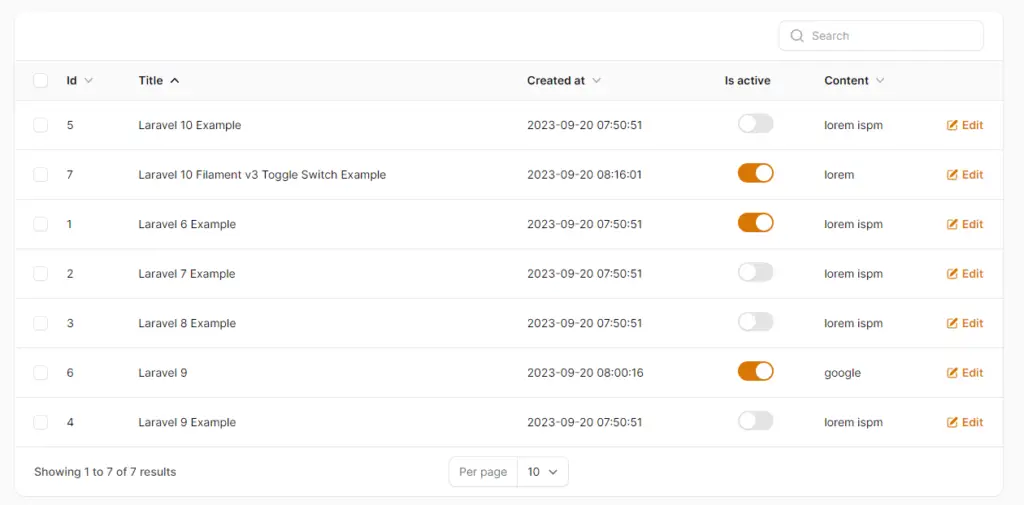