In this section, we will create a CRUD (Create, Read, Update, and Delete) app using Laravel 10 with FilamentPHP v3.
Step 1: Install Laravel & Connect Database
Run below command to create laravel project.
composer create-project laravel/laravel filamentphp-crud
Now, you need to connect the Laravel app to the database. Open the .env configuration file and add the database credentials as suggested below.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_user_name
DB_PASSWORD=database_password
Step 2: Install Laravel Filament
Install the Filament Panel Builder by running the following commands in your Laravel project directory.
composer require filament/filament:"^3" -W
php artisan filament:install --panels
Create a filament user for admin.
php artisan make:filament-user
Give username, email and password.
Name:
> admin
Email address:
> [email protected]
Password:
>
Success! [email protected] may now log in at http://localhost/admin/login.
Step 3: Login Filament admin
http://localhost:8000/admin/login
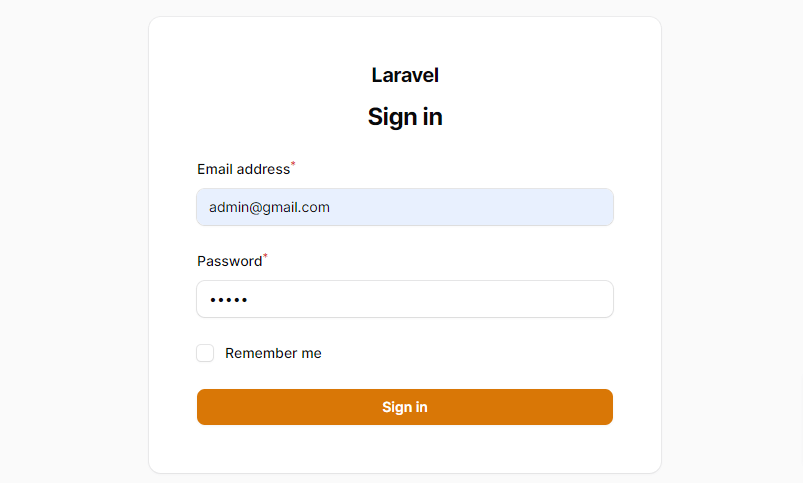
Step 4: Create Blog Model and Migration
Run below command to create Blog modal and migration.
php artisan make:model Blog -m
create_blogs_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('blogs', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('blogs');
}
};
App/Modal/Blog.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Blog extends Model
{
use HasFactory;
protected $fillable = [
'title',
'content'
];
}
run migration
php artisan migrate
Step 5: Create a Model Resource
Run below to command create a resource for the App\Models\Blog model.
php artisan make:filament-resource Blog
This will create several files in the app/Filament/Resources directory.
+-- BlogResource.php
+-- CustomerResource
| +-- Pages
| | +-- CreateBlog.php
| | +-- EditBlog.php
| | +-- ListBlogs.php
Step 6: Create a Blog Filament CRUD
Filament/Resources/BlogResource.php
<?php
namespace App\Filament\Resources;
use App\Filament\Resources\BlogResource\Pages;
use App\Filament\Resources\BlogResource\RelationManagers;
use App\Models\Blog;
use Filament\Forms;
use Filament\Forms\Form;
use Filament\Resources\Resource;
use Filament\Tables;
use Filament\Tables\Table;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Components\Textarea;
use Filament\Tables\Columns\TextColumn;
use Filament\Forms\Components\Section;
use Illuminate\Database\Eloquent\Builder;
use Illuminate\Database\Eloquent\SoftDeletingScope;
class BlogResource extends Resource
{
protected static ?string $model = Blog::class;
protected static ?string $navigationIcon = 'heroicon-o-rectangle-stack';
public static function form(Form $form): Form
{
return $form
->schema([
Section::make()
->schema([
TextInput::make('title')->required(),
Textarea::make('content')->required(),
])
]);
}
public static function table(Table $table): Table
{
return $table
->columns([
TextColumn::make('id'),
TextColumn::make('title'),
TextColumn::make('content'),
])
->filters([
//
])
->actions([
Tables\Actions\EditAction::make(),
])
->bulkActions([
Tables\Actions\BulkActionGroup::make([
Tables\Actions\DeleteBulkAction::make(),
]),
])
->emptyStateActions([
Tables\Actions\CreateAction::make(),
]);
}
public static function getRelations(): array
{
return [
//
];
}
public static function getPages(): array
{
return [
'index' => Pages\ListBlogs::route('/'),
'create' => Pages\CreateBlog::route('/create'),
'edit' => Pages\EditBlog::route('/{record}/edit'),
];
}
}
Creating Operations in Laravel 10 with FilamentPHP.
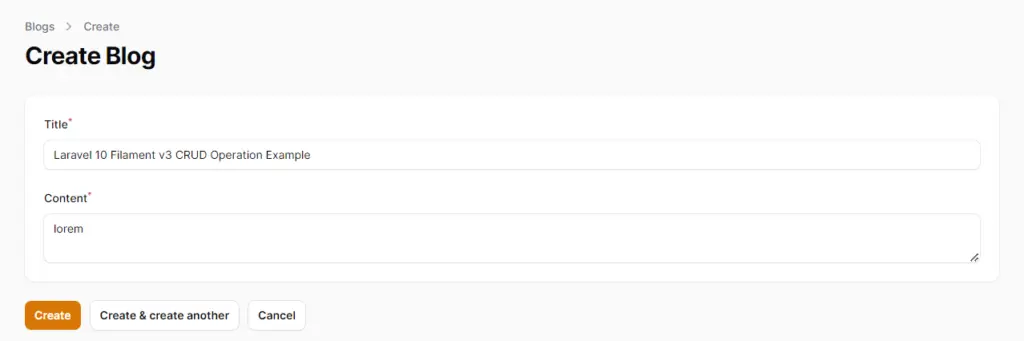
Laravel 10 with filamentphp blogs index.
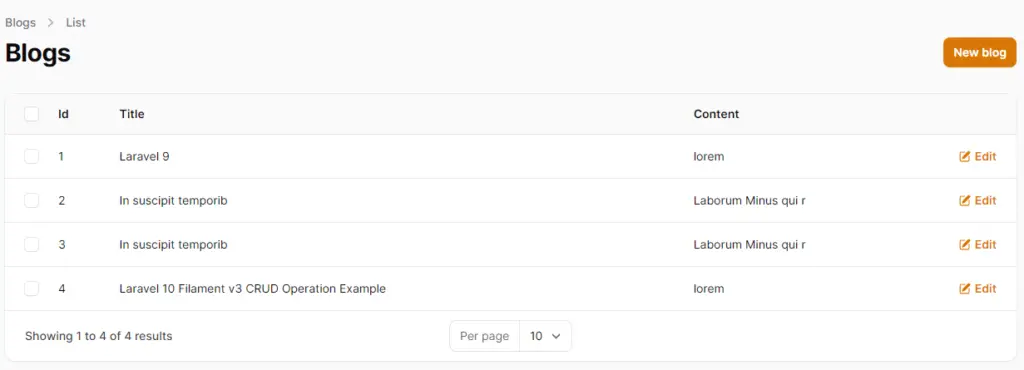
Editing and Deleting Blogs in Laravel 10 with FilamentPHP.
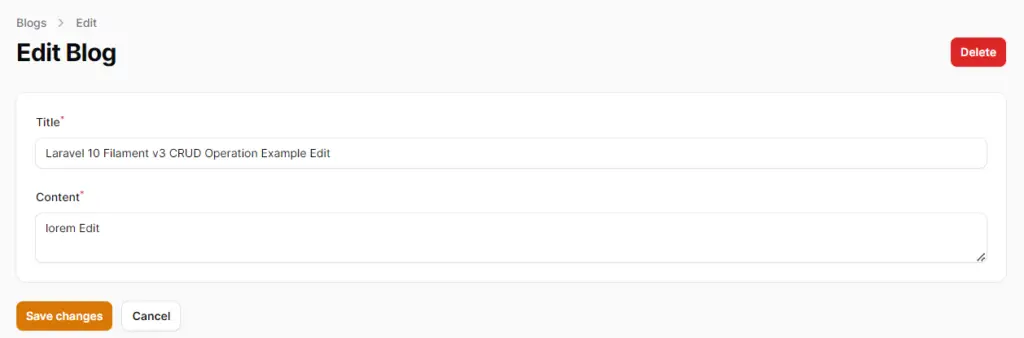