In this tutorial, we’ll create a 404 error page in React.js using Tailwind CSS. We’ll cover examples such as a 404 page with React Router integration and a 404 page featuring an image, all styled with Tailwind CSS for a visually appealing result.
Tool Use
React JS
Tailwind CSS
React router
First you need to setup react project with tailwind css. You can install manually or you read below blog.
How to install Tailwind CSS in React
Install & Setup Vite + React + Typescript + Tailwind CSS 3
Yo can read how to use 404 page using react router in react.
https://reactrouter.com/en/main/start/tutorial#handling-not-found-errors
Example 1
React with tailwind css 404 page with react router.
import { Link, useRouteError } from "react-router-dom";
export default function ErrorPage() {
const error = useRouteError();
console.error(error);
return (
<>
<div className="flex justify-center items-center h-screen bg-purple-600">
<div id="error-page">
<h1 className="lg:text-6xl font-bold text-2xl text-white">Oops!</h1>
<p className="text-xl text-white">
Sorry, an unexpected error has occurred.
</p>
<p className="text-3xl text-white">
{error.statusText || error.message}
</p>
<div className="mt-4">
<Link
to="/"
className="px-5 py-2 bg-white rounded-md hover:bg-gray-100"
>
Home
</Link>
</div>
</div>
</div>
</>
);
}
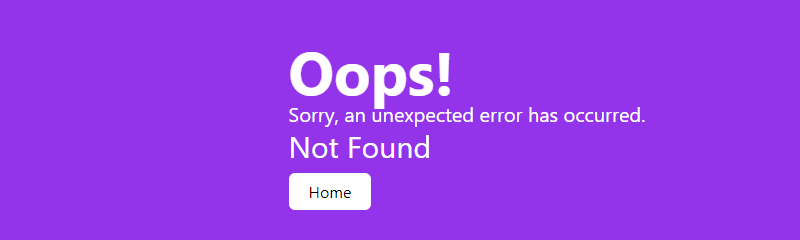
React with tailwind css 404 page message with react router.
import { Link, useRouteError } from "react-router-dom";
export default function ErrorPage() {
const error = useRouteError();
console.error(error);
return (
<>
<div className="flex items-center justify-center h-screen">
<div className="bg-white">
<div className="flex flex-col items-center">
<h1 className="font-bold text-3xl text-blue-600 lg:text-6xl">
404
</h1>
<h6 className="mb-2 text-2xl font-bold text-center text-gray-800 md:text-3xl">
<span className="text-red-500">Oops!</span> Page{" "}
{error.statusText}
</h6>
<p className="mb-4 text-center text-gray-500 md:text-lg">
The page you’re looking for doesn’t exist.
</p>
<Link
to="/"
className="px-5 py-2 rounded-md text-blue-100 bg-blue-600 hover:bg-blue-700"
>
Go home
</Link>
</div>
</div>
</div>
</>
);
}
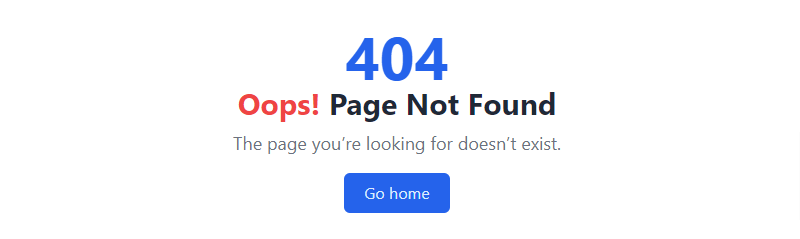
Example 2
React with tailwind css 404 page with image.
import { Link, useRouteError } from "react-router-dom";
export default function ErrorPage() {
const error = useRouteError();
console.error(error);
return (
<>
<div className="flex items-center justify-center w-screen h-screen">
<div className="px-4 lg:py-12">
<div className="lg:gap-4 lg:flex">
<div className="flex flex-col items-center justify-center md:py-24 lg:py-32">
<h1 className="font-bold text-blue-600 text-9xl">404</h1>
<p className="mb-2 text-2xl font-bold text-center text-gray-800 md:text-3xl">
<span className="text-red-500">Oops!</span> Page{" "}
{error.statusText}
</p>
<p className="mb-8 text-center text-gray-500 md:text-lg">
The page you’re looking for doesn’t exist.
</p>
<Link
to="/"
className="px-5 py-2 rounded-md text-blue-100 bg-blue-600 hover:bg-blue-700"
>
Go home
</Link>
</div>
<div className="mt-4">
<img
src="https://cdn.pixabay.com/photo/2016/11/22/23/13/black-dog-1851106__340.jpg"
alt="img"
className="object-cover w-full h-full"
/>
</div>
</div>
</div>
</div>
</>
);
}