In this tutorial, we’ll create dropdown menus in React using Tailwind CSS. We’ll cover various aspects, including building a dropdown menu in React with Tailwind and implementing a custom select dropdown using Headless UI and Tailwind CSS.
Tool Use
React JS
Tailwind CSS
Headless UI
First you need to setup react project with tailwind css. You can install manually or you read below blog.
How to install Tailwind CSS in React
Install & Setup Vite + React + Typescript + Tailwind CSS 3
Example 1
Building Simple Dropdown Menu Items with Tailwind and React.js.
import React from "react";
export default function DropdownComponent() {
return (
<div className="relative w-full lg:max-w-sm">
<select className="w-full p-2.5 text-gray-500 bg-white border rounded-md shadow-sm outline-none appearance-none focus:border-indigo-600">
<option>ReactJS Dropdown</option>
<option>Laravel 9 with React</option>
<option>React with Tailwind CSS</option>
<option>React With Headless UI</option>
</select>
</div>
);
}
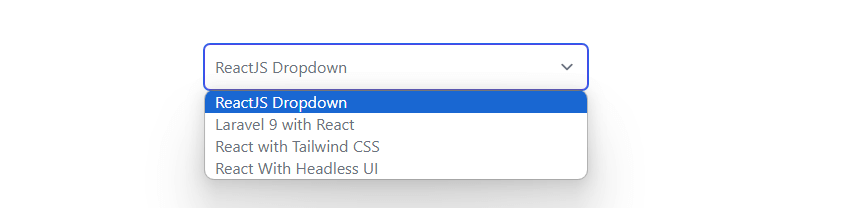
Example 2
Creating a Disabled Dropdown Menu with Selected Item in React and Tailwind CSS.
import React from "react";
export default function DropdownComponent() {
return (
<div className="relative w-full lg:max-w-sm">
<select
disabled
className="w-full p-2.5 text-gray-500 bg-white border rounded-md shadow-sm outline-none appearance-none focus:border-indigo-600"
>
<option>ReactJS Dropdown</option>
<option>Laravel 9 with React</option>
<option>React with Tailwind CSS</option>
<option selected>React With Headless UI</option>
</select>
</div>
);
}
Example 3
Designing a Dropdown Menu with SVG Icons in React and Tailwind CSS, Featuring Heroicons.
import React from "react";
export default function DropdownComponent() {
return (
<div className="inline-flex bg-white border rounded-md">
<a
href="#"
className="px-4 py-2 text-sm text-gray-600 hover:text-gray-700 hover:bg-gray-50 rounded-l-md"
>
Option
</a>
<div className="relative">
<button
type="button"
className="inline-flex items-center justify-center h-full px-2 text-gray-600 border-l border-gray-100 hover:text-gray-700 rounded-r-md hover:bg-gray-50"
>
<svg
xmlns="http://www.w3.org/2000/svg"
className="w-4 h-4"
fill="none"
viewBox="0 0 24 24"
stroke="currentColor"
strokeWidth={2}
>
<path
strokeLinecap="round"
strokeLinejoin="round"
d="M19 9l-7 7-7-7"
/>
</svg>
</button>
<div className="absolute right-0 z-10 w-56 mt-4 origin-top-right bg-white border border-gray-100 rounded-md shadow-lg">
<div className="p-2">
<a
href="#"
className="block px-4 py-2 text-sm text-gray-500 rounded-lg hover:bg-gray-50 hover:text-gray-700"
>
ReactJS Dropdown 1
</a>
<a
href="#"
className="block px-4 py-2 text-sm text-gray-500 rounded-lg hover:bg-gray-50 hover:text-gray-700"
>
ReactJS Dropdown 2
</a>
<a
href="#"
className="block px-4 py-2 text-sm text-gray-500 rounded-lg hover:bg-gray-50 hover:text-gray-700"
>
ReactJS Dropdown 3
</a>
</div>
</div>
</div>
</div>
);
}
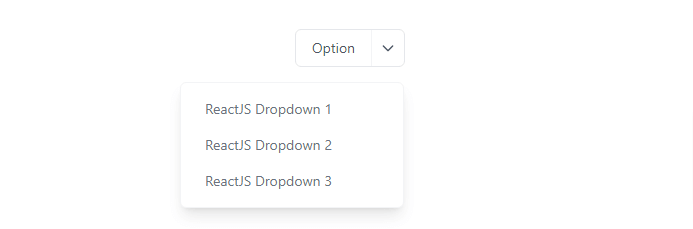
Example 4
Creating a Dropdown Select Menu in React.js with Tailwind CSS, Leveraging Headless UI.
Install Headless UI
To get started, install Headless UI via npm:
npm install @headlessui/react
import { Fragment } from "react";
import { Menu, Transition } from "@headlessui/react";
function classNames(...classes) {
return classes.filter(Boolean).join(" ");
}
export default function DropdownComponent() {
return (
<Menu as="div" className="relative inline-block text-left">
<div>
<Menu.Button className="inline-flex justify-center w-full px-4 py-2 text-sm font-medium text-gray-700 bg-white border border-gray-300 rounded-md shadow-sm hover:bg-gray-50 focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-offset-gray-100 focus:ring-indigo-500">
Options
<svg
xmlns="http://www.w3.org/2000/svg"
className="w-5 h-5 ml-2 -mr-1"
fill="none"
viewBox="0 0 24 24"
stroke="currentColor"
strokeWidth={2}
>
<path
strokeLinecap="round"
strokeLinejoin="round"
d="M19 9l-7 7-7-7"
/>
</svg>
</Menu.Button>
</div>
<Transition
as={Fragment}
enter="transition ease-out duration-100"
enterFrom="transform opacity-0 scale-95"
enterTo="transform opacity-100 scale-100"
leave="transition ease-in duration-75"
leaveFrom="transform opacity-100 scale-100"
leaveTo="transform opacity-0 scale-95"
>
<Menu.Items className="absolute right-0 w-56 mt-2 origin-top-right bg-white rounded-md shadow-lg ring-1 ring-black ring-opacity-5 focus:outline-none">
<div className="py-1">
<Menu.Item>
{({ active }) => (
<a
href="#"
className={classNames(
active
? "bg-gray-100 text-gray-900"
: "text-gray-700",
"block px-4 py-2 text-sm"
)}
>
Account settings
</a>
)}
</Menu.Item>
<Menu.Item>
{({ active }) => (
<a
href="#"
className={classNames(
active
? "bg-gray-100 text-gray-900"
: "text-gray-700",
"block px-4 py-2 text-sm"
)}
>
Support
</a>
)}
</Menu.Item>
<Menu.Item>
{({ active }) => (
<a
href="#"
className={classNames(
active
? "bg-gray-100 text-gray-900"
: "text-gray-700",
"block px-4 py-2 text-sm"
)}
>
License
</a>
)}
</Menu.Item>
<form method="POST" action="#">
<Menu.Item>
{({ active }) => (
<button
type="submit"
className={classNames(
active
? "bg-gray-100 text-gray-900"
: "text-gray-700",
"block w-full text-left px-4 py-2 text-sm"
)}
>
Sign out
</button>
)}
</Menu.Item>
</form>
</div>
</Menu.Items>
</Transition>
</Menu>
);
}