Build Interactive Hover Menus in React with Tailwind CSS. Learn to create menus that activate on hover and display a hand cursor for a user-friendly experience.
Tool Use
React JS
Tailwind CSS
Heroicons Icon
First you need to setup react project with tailwind css. You can install manually or you read below blog.
How to install Tailwind CSS in React
Install & Setup Vite + React + Typescript + Tailwind CSS 3
Example 1
Create Hover Menus in React with Tailwind CSS. This tutorial uses onMouseOver, onMouseLeave, and useState for interactive menus.
import { useState } from "react";
export default function HoverDropdown() {
const [open, setOpen] = useState(false);
return (
<div className="flex justify-center mt-20">
<div onMouseLeave={() => setOpen(false)} className="relative">
<button
onMouseOver={() => setOpen(true)}
className="flex items-center p-2 bg-white border rounded-md"
>
<span className="mr-4">Dropdown Button</span>
</button>
<ul
className={`absolute right-0 w-40 py-2 mt-2 rounded-lg shadow-xl ${
open ? "block" : "hidden"
}`}
>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 1
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 2
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 3
</li>
</ul>
</div>
</div>
);
}
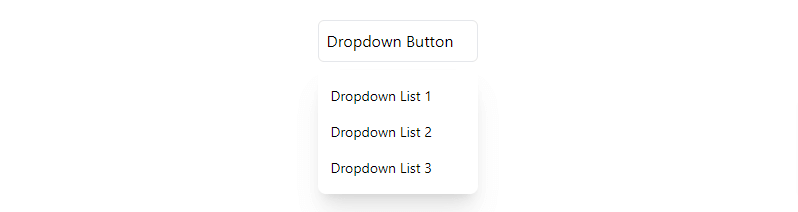
React tailwind on hover open dropdown menu with Heroicons dropdown Icon.
import { useState } from "react";
export default function HoverDropdown() {
const [open, setOpen] = useState(false);
return (
<div className="flex justify-center mt-20">
<div onMouseLeave={() => setOpen(false)} className="relative">
<button
onMouseOver={() => setOpen(true)}
className="flex items-center p-2 bg-white border rounded-md"
>
<span className="mr-2">Dropdown Button</span>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" strokeWidth={1.5} stroke="currentColor" className="w-4 h-4">
<path strokeLinecap="round" strokeLinejoin="round" d="M19.5 8.25l-7.5 7.5-7.5-7.5" />
</svg>
</button>
<ul
className={`absolute right-0 w-40 py-2 mt-2 rounded-lg shadow-xl ${
open ? "block" : "hidden"
}`}
>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 1
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 2
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 3
</li>
</ul>
</div>
</div>
);
}

Example 2
React tailwind hoverable menu using only svg icon.
import { useState } from "react";
export default function HoverDropdown() {
const [open, setOpen] = useState(false);
return (
<div className="flex justify-center mt-20">
<div onMouseLeave={() => setOpen(false)} className="relative">
<button onMouseOver={() => setOpen(true)} className="flex items-center p-2 rounded-md border-blue-600">
<svg
xmlns="http://www.w3.org/2000/svg"
fill="none"
viewBox="0 0 24 24"
strokeWidth={1.5}
stroke="currentColor"
className="w-6 h-6"
>
<path
strokeLinecap="round"
strokeLinejoin="round"
d="M12 6.75a.75.75 0 110-1.5.75.75 0 010 1.5zM12 12.75a.75.75 0 110-1.5.75.75 0 010 1.5zM12 18.75a.75.75 0 110-1.5.75.75 0 010 1.5z"
/>
</svg>
</button>
<ul
className={`absolute right-0 w-40 py-2 mt-2 rounded-lg shadow-xl ${
open ? "block" : "hidden"
}`}
>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 1
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 2
</li>
<li className="flex w-full items-center px-3 py-2 text-sm hover:bg-gray-100">
Dropdown List 3
</li>
</ul>
</div>
</div>
);
}
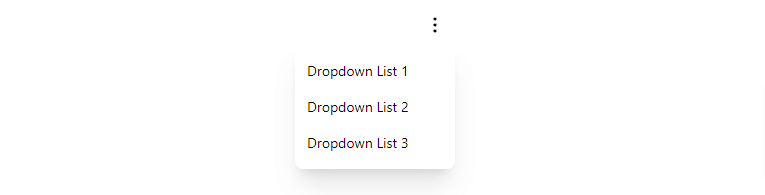