Designing an engaging chat interface is easy with Tailwind CSS. In this guide, we’ll show you how to use Tailwind’s utility classes to build a sleek and responsive Chat UI. Perfect for any modern web application, this tutorial will help you create a chat experience that looks great and works seamlessly. Let’s get started!
Simple Chat UI
<div class="flex flex-col h-screen bg-gray-100">
<!-- Chat header -->
<div class="bg-white shadow-md">
<div class="max-w-3xl mx-auto py-3 px-4 flex items-center justify-between">
<div class="flex items-center space-x-4">
<img src="https://via.placeholder.com/40" alt="Chat avatar" class="w-10 h-10 rounded-full">
<div>
<h1 class="text-xl font-semibold text-gray-800">Chat UI Example</h1>
<p class="text-sm text-gray-500">Online</p>
</div>
</div>
<button class="text-gray-500 hover:text-gray-700">
<svg xmlns="http://www.w3.org/2000/svg" class="h-6 w-6" fill="none" viewBox="0 0 24 24" stroke="currentColor">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M12 5v.01M12 12v.01M12 19v.01M12 6a1 1 0 110-2 1 1 0 010 2zm0 7a1 1 0 110-2 1 1 0 010 2zm0 7a1 1 0 110-2 1 1 0 010 2z" />
</svg>
</button>
</div>
</div>
<!-- Chat messages -->
<div class="flex-1 overflow-y-auto p-4 space-y-6 bg-gray-50">
<!-- Received message -->
<div class="flex items-start space-x-2">
<img src="https://via.placeholder.com/40" alt="User avatar" class="w-8 h-8 rounded-full">
<div class="flex flex-col">
<div class="bg-white p-3 rounded-lg rounded-tl-none shadow-sm max-w-xs lg:max-w-md">
<p class="text-sm text-gray-800">Hi there! How can I help you today?</p>
</div>
<span class="text-xs text-gray-500 mt-1">10:23 AM</span>
</div>
</div>
<!-- Sent message -->
<div class="flex items-start justify-end space-x-2">
<div class="flex flex-col items-end">
<div class="bg-blue-500 p-3 rounded-lg rounded-tr-none shadow-sm max-w-xs lg:max-w-md">
<p class="text-sm text-white">Hello! I have a question about your services.</p>
</div>
<span class="text-xs text-gray-500 mt-1">10:24 AM</span>
</div>
<img src="https://via.placeholder.com/40" alt="My avatar" class="w-8 h-8 rounded-full">
</div>
</div>
<!-- Chat input -->
<div class="bg-white border-t-2 border-gray-200 px-4 pt-4 mb-2 sm:mb-0">
<div class="relative flex">
<span class="absolute inset-y-0 flex items-center">
<button type="button" class="inline-flex items-center justify-center rounded-full h-12 w-12 transition duration-500 ease-in-out text-gray-500 hover:bg-gray-300 focus:outline-none">
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke="currentColor" class="h-6 w-6 text-gray-600">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M19 11a7 7 0 01-7 7m0 0a7 7 0 01-7-7m7 7v4m0 0H8m4 0h4m-4-8a3 3 0 01-3-3V5a3 3 0 116 0v6a3 3 0 01-3 3z" />
</svg>
</button>
</span>
<input type="text" placeholder="Write your message!" class="w-full focus:outline-none focus:placeholder-gray-400 text-gray-600 placeholder-gray-600 pl-12 bg-gray-200 rounded-md py-3">
<div class="absolute right-0 items-center inset-y-0 hidden sm:flex">
<button type="button" class="inline-flex items-center justify-center rounded-lg px-4 py-3 transition duration-500 ease-in-out text-white bg-blue-500 hover:bg-blue-400 focus:outline-none">
<span class="font-bold">Send</span>
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 20 20" fill="currentColor" class="h-6 w-6 ml-2 transform rotate-90">
<path d="M10.894 2.553a1 1 0 00-1.788 0l-7 14a1 1 0 001.169 1.409l5-1.429A1 1 0 009 15.571V11a1 1 0 112 0v4.571a1 1 0 00.725.962l5 1.428a1 1 0 001.17-1.408l-7-14z"></path>
</svg>
</button>
</div>
</div>
</div>
</div>
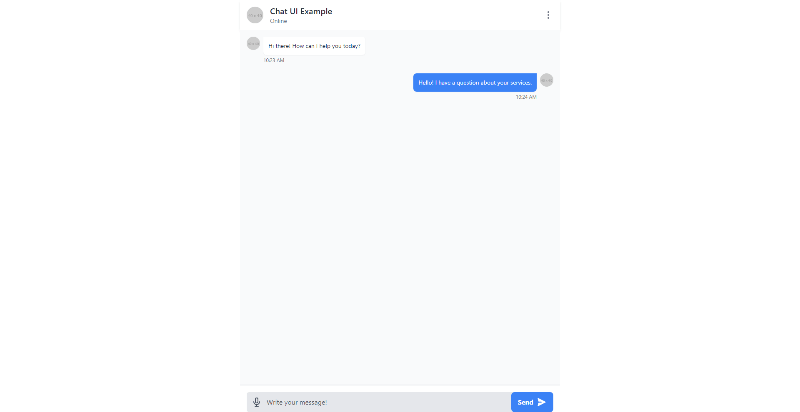
Group Chat UI
<div class="flex h-screen antialiased text-gray-800">
<div class="flex flex-row h-full w-full overflow-x-hidden">
<!-- Sidebar -->
<div class="flex flex-col py-8 pl-6 pr-2 w-64 bg-white flex-shrink-0">
<div class="flex flex-row items-center justify-center h-12 w-full">
<div class="flex items-center justify-center rounded-2xl text-indigo-700 bg-indigo-100 h-10 w-10">
<svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M8 10h.01M12 10h.01M16 10h.01M9 16H5a2 2 0 01-2-2V6a2 2 0 012-2h14a2 2 0 012 2v8a2 2 0 01-2 2h-5l-5 5v-5z"></path>
</svg>
</div>
<div class="ml-2 font-bold text-2xl">QuickChat</div>
</div>
<div class="flex flex-col mt-8">
<div class="flex flex-row items-center justify-between text-xs">
<span class="font-bold">Active Conversations</span>
<span class="flex items-center justify-center bg-gray-300 h-4 w-4 rounded-full">4</span>
</div>
<div class="flex flex-col space-y-1 mt-4 -mx-2 h-48 overflow-y-auto">
<button class="flex flex-row items-center hover:bg-gray-100 rounded-xl p-2">
<div class="flex items-center justify-center h-8 w-8 bg-indigo-200 rounded-full">
H
</div>
<div class="ml-2 text-sm font-semibold">Henry Boyd</div>
</button>
<!-- More user buttons here -->
</div>
</div>
</div>
<!-- Chat Area -->
<div class="flex flex-col flex-auto h-full p-6">
<div class="flex flex-col flex-auto flex-shrink-0 rounded-2xl bg-gray-100 h-full p-4">
<div class="flex flex-col h-full overflow-x-auto mb-4">
<div class="flex flex-col h-full">
<div class="grid grid-cols-12 gap-y-2">
<div class="col-start-1 col-end-8 p-3 rounded-lg">
<div class="flex flex-row items-center">
<div class="flex items-center justify-center h-10 w-10 rounded-full bg-indigo-500 flex-shrink-0">
A
</div>
<div class="relative ml-3 text-sm bg-white py-2 px-4 shadow rounded-xl">
<div>Hey How are you today?</div>
</div>
</div>
</div>
<!-- More messages here -->
</div>
</div>
</div>
<div class="flex flex-row items-center h-16 rounded-xl bg-white w-full px-4">
<div>
<button class="flex items-center justify-center text-gray-400 hover:text-gray-600">
<svg class="w-5 h-5" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M15.172 7l-6.586 6.586a2 2 0 102.828 2.828l6.414-6.586a4 4 0 00-5.656-5.656l-6.415 6.585a6 6 0 108.486 8.486L20.5 13"></path>
</svg>
</button>
</div>
<div class="flex-grow ml-4">
<div class="relative w-full">
<input type="text" class="flex w-full border rounded-xl focus:outline-none focus:border-indigo-300 pl-4 h-10"/>
<button class="absolute flex items-center justify-center h-full w-12 right-0 top-0 text-gray-400 hover:text-gray-600">
<svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M14.828 14.828a4 4 0 01-5.656 0M9 10h.01M15 10h.01M21 12a9 9 0 11-18 0 9 9 0 0118 0z"></path>
</svg>
</button>
</div>
</div>
<div class="ml-4">
<button class="flex items-center justify-center bg-indigo-500 hover:bg-indigo-600 rounded-xl text-white px-4 py-1 flex-shrink-0">
<span>Send</span>
<span class="ml-2">
<svg class="w-4 h-4 transform rotate-45 -mt-px" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M12 19l9 2-9-18-9 18 9-2zm0 0v-8"></path>
</svg>
</span>
</button>
</div>
</div>
</div>
</div>
</div>
</div>
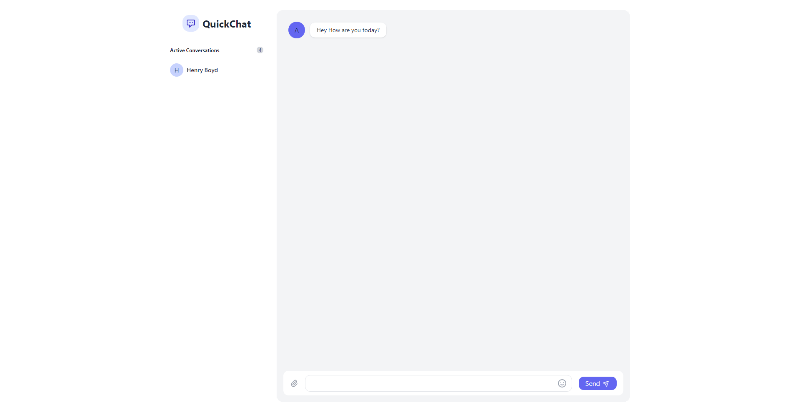
Direct Message UI with Dark Mode
<div class="flex h-screen antialiased text-gray-800">
<div class="flex flex-col flex-shrink-0 w-64 bg-gray-900 text-gray-300">
<div class="flex items-center justify-center h-14 border-b border-gray-800">
<h1 class="text-xl font-bold">Messages</h1>
</div>
<div class="overflow-y-auto">
<div class="flex items-center px-4 py-3 hover:bg-gray-800">
<div class="relative">
<img class="w-10 h-10 rounded-full" src="https://via.placeholder.com/40" alt="User avatar">
<div class="absolute bottom-0 right-0 w-3 h-3 bg-green-400 border-2 border-gray-900 rounded-full"></div>
</div>
<div class="ml-3">
<div class="font-semibold">John Doe</div>
<div class="text-sm text-gray-500">Online</div>
</div>
</div>
<!-- More user list items here -->
</div>
</div>
<div class="flex flex-col flex-auto bg-gray-800">
<div class="flex flex-col flex-auto flex-shrink-0 h-full p-4">
<div class="flex flex-col h-full overflow-x-auto mb-4">
<div class="flex flex-col h-full">
<div class="grid grid-cols-12 gap-y-2">
<div class="col-start-1 col-end-8 p-3 rounded-lg">
<div class="flex flex-row items-center">
<div class="flex items-center justify-center h-10 w-10 rounded-full bg-indigo-500 flex-shrink-0">
J
</div>
<div class="relative ml-3 text-sm bg-gray-700 py-2 px-4 shadow rounded-xl">
<div class="text-gray-200">Hey, how's it going?</div>
</div>
</div>
</div>
<div class="col-start-6 col-end-13 p-3 rounded-lg">
<div class="flex items-center justify-start flex-row-reverse">
<div class="flex items-center justify-center h-10 w-10 rounded-full bg-indigo-500 flex-shrink-0">
Y
</div>
<div class="relative mr-3 text-sm bg-indigo-600 py-2 px-4 shadow rounded-xl">
<div class="text-white">I'm good, thanks! How about you?</div>
<div class="absolute text-xs bottom-0 right-0 -mb-5 mr-2 text-gray-500">Seen</div>
</div>
</div>
</div>
<!-- More messages here -->
</div>
</div>
</div>
<div class="flex flex-row items-center h-16 rounded-xl bg-gray-700 w-full px-4">
<div>
<button class="flex items-center justify-center text-gray-400 hover:text-gray-200">
<svg class="w-5 h-5" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M15.172 7l-6.586 6.586a2 2 0 102.828 2.828l6.414-6.586a4 4 0 00-5.656-5.656l-6.415 6.585a6 6 0 108.486 8.486L20.5 13"></path>
</svg>
</button>
</div>
<div class="flex-grow ml-4">
<div class="relative w-full">
<input type="text" class="flex w-full border rounded-xl focus:outline-none focus:border-indigo-300 pl-4 h-10 bg-gray-600 text-gray-200"/>
<button class="absolute flex items-center justify-center h-full w-12 right-0 top-0 text-gray-400 hover:text-gray-200">
<svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M14.828 14.828a4 4 0 01-5.656 0M9 10h.01M15 10h.01M21 12a9 9 0 11-18 0 9 9 0 0118 0z"></path>
</svg>
</button>
</div>
</div>
<div class="ml-4">
<button class="flex items-center justify-center bg-indigo-500 hover:bg-indigo-600 rounded-xl text-white px-4 py-1 flex-shrink-0">
<span>Send</span>
<span class="ml-2">
<svg class="w-4 h-4 transform rotate-45 -mt-px" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M12 19l9 2-9-18-9 18 9-2zm0 0v-8"></path>
</svg>
</span>
</button>
</div>
</div>
</div>
</div>
</div>
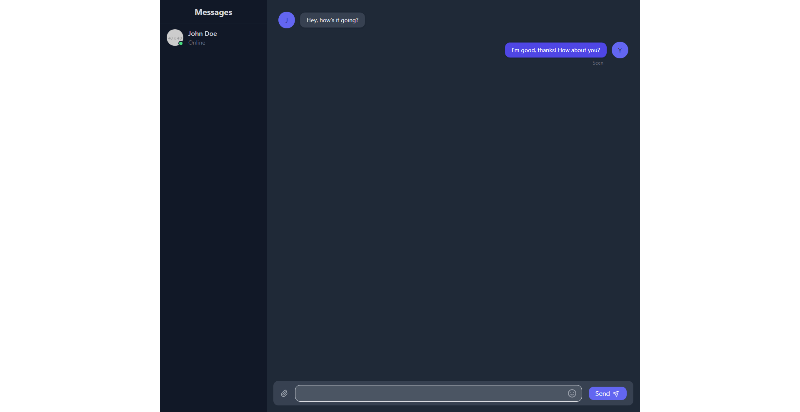