In this section, we will explore how to create a REST API CRUD application in Laravel 9. This tutorial will guide you through building a simple yet clean Laravel API CRUD using Requests for validation and Resources for displaying important and organized data. We will focus on creating a well-structured and maintainable Laravel 9 API CRUD application.
Step 1: Install Laravel & Connect Database
Installing a fresh new laravel 9 application, so head over to the terminal, type the command, and create a new laravel app.
composer create-project laravel/laravel crud
Now, you have to connect the laravel app to the database, hence open the .env configuration file and add the database credentials as suggested below.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_user_name
DB_PASSWORD=database_password
Step 2: Create Model, Request, Resource, API Controller, and Route
Create Post Modal with migration.
php artisan make:model Post -m
Create Post Request for validation.
php artisan make:request StorePostRequest
Create Post Resource for show clean data.
php artisan make:resource PostResource
Create Post Api controller.
php artisan make:controller Api/PostController --model=Post
create_posts_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
};
app/Http/Models/Post.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
use HasFactory;
protected $fillable = ['title', 'description'];
}
app/Http/Requests/StorePostRequest.php
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return false;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'title' => ['required', 'max:70'],
'description' => ['required']
];
}
}
app/Http/Controllers/Api/PostController.php
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Http\Requests\StorePostRequest;
use App\Http\Resources\PostResource;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$posts = Post::all();
return PostResource::collection($posts);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(StorePostRequest $request)
{
$posts = Post::create($request->all());
return new PostResource($posts);
}
/**
* Display the specified resource.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function show(Post $post)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function edit(Post $post)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function update(StorePostRequest $request, Post $post)
{
$post->update($request->all());
return new PostResource($post);
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function destroy(Post $post)
{
$post->delete();
return response(null, 204);
}
}
routes/api.php
<?php
use App\Http\Controllers\Api\PostController;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
Route::apiResource('posts',PostController::class);
Run Laravel App.
php artisan serve
Step 3: Test CRUD App in Postman API
POST method
http://localhost:8000/api/posts
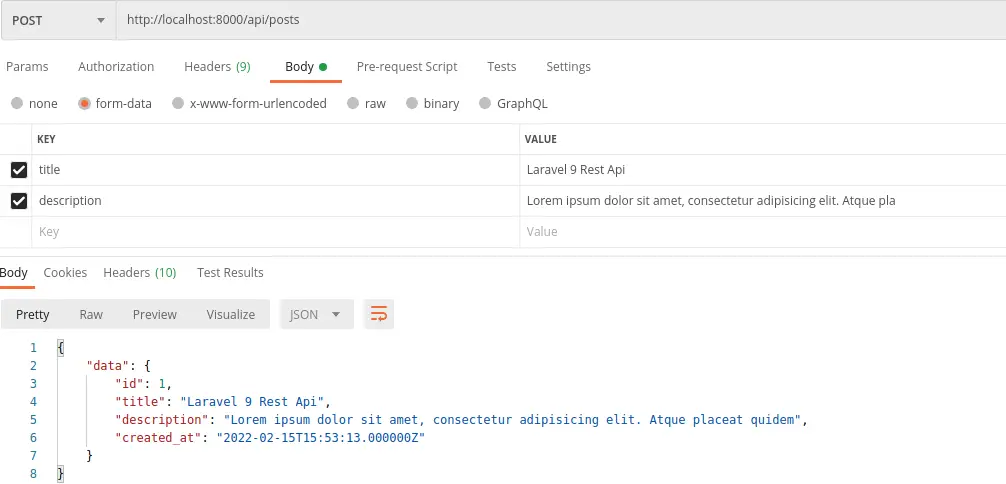
GET method
http://localhost:8000/api/posts
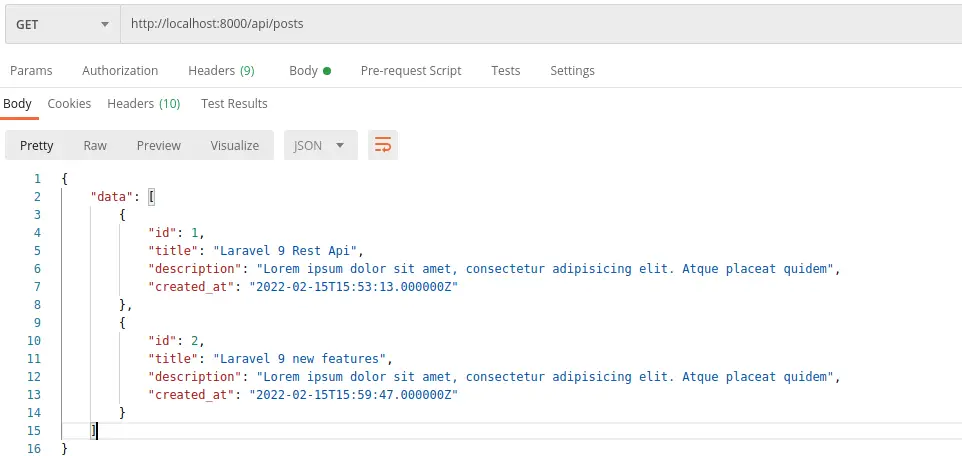
PUT method
http://localhost:8000/api/posts/1
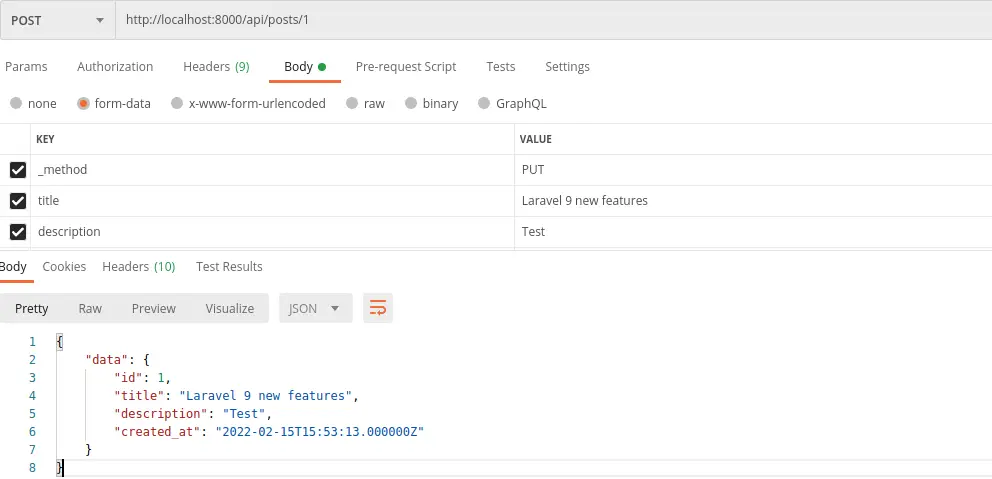
DELETE method
http://localhost:8000/api/posts/1
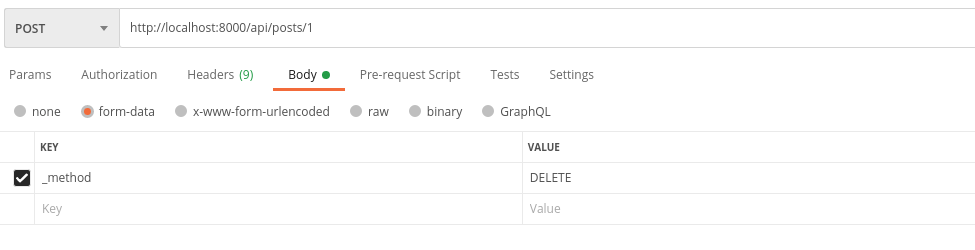