In this section, we’ll learn how to upload image files with validation in Laravel 9. Laravel provides its own file storage system, eliminating the need to install third-party packages. We’ll be using Tailwind CSS 3 along with Laravel 9 for this tutorial.
Step 1: Create Laravel Project
To install a fresh new Laravel 9 application, navigate to your terminal, type the command, and create a new Laravel app.
composer create-project laravel/laravel project-name
Step 2: Setup Databases
Now, you need to connect the Laravel app to the database. Open the .env configuration file and add the database credentials as suggested below.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_user_name
DB_PASSWORD=database_password
Step 3: Create Image Model, Migration, Controller, and Routes
Run shorthand command to create image modal, migration and controller.
php artisan make:model Image -mc
images_table.php
public function up()
{
Schema::create('images', function (Blueprint $table) {
$table->id();
$table->string('image');
$table->timestamps();
});
}
app/Models/Image.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Image extends Model
{
use HasFactory;
protected $fillable = [
'image'
];
}
app/Http/Controllers/ImageController.php
<?php
namespace App\Http\Controllers;
use App\Models\Image;
use Illuminate\Http\Request;
class ImageController extends Controller
{
public function index()
{
return view('image');
}
public function store(Request $request)
{
$this->validate($request, [
'image' => 'required|image|mimes:jpg,png,jpeg,gif,svg|max:2048',
]);
$image_path = $request->file('image')->store('image', 'public');
$data = Image::create([
'image' => $image_path,
]);
session()->flash('success', 'Image Upload successfully');
return redirect()->route('image.index');
}
}
Create image routes.
<?php
use App\Http\Controllers\ImageController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/image', [ImageController::class,'index'])->name('image.index');
Route::post('/image', [ImageController::class,'store'])->name('image.store');
Step 4: Create Blade File
views/image.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>image Upload in Laravel 9</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body>
<div class="relative flex items-center min-h-screen justify-center overflow-hidden">
<form action="{{ route('image.store') }}" method="POST" class="shadow p-12" enctype="multipart/form-data">
@csrf
<label class="block mb-4">
<span class="sr-only">Choose File</span>
<input type="file" name="image"
class="block w-full text-sm text-gray-500 file:mr-4 file:py-2 file:px-4 file:rounded-full file:border-0 file:text-sm file:font-semibold file:bg-blue-50 file:text-blue-700 hover:file:bg-blue-100" />
@error('image')
<span class="text-red-600 text-sm">{{ $message }}</span>
@enderror
</label>
<button type="submit" class="px-4 py-2 text-sm text-white bg-indigo-600 rounded">Submit</button>
</form>
</div>
</body>
</html>
Step 5: Migrate database & Storage links
run migrate database.
php artisan migrate
Storage links to public directory.
php artisan storage:link
Step 6: Run the Server
php artisan serve
http://localhost:8000/image
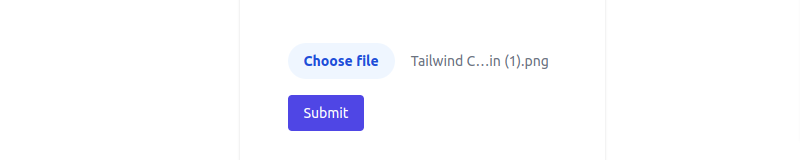