In this tutorial, we’ll demonstrate how to enable email verification using the Laravel UI package. Laravel UI simplifies authentication scaffolding, providing a straightforward UI that’s easy to install. If you’re using Bootstrap UI, this package is a great choice.
Step 1: Set up Configuring the SMTP Server
You need to add SMTP credentials in the .env file. In this section, I’m using Mailtrap, but you can use any mail service. Simply register for a Mailtrap account, navigate to the index page, find Integrations, select the Laravel section, and add the provided credentials to your .env file.
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=<YOUR_MAILTRAP_USERNAME>
MAIL_PASSWORD=<YOUR_MAILTRAP_PASSWORD>
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=<YOUR_Email_Address>
MAIL_FROM_NAME="${APP_NAME}"
Step 2: Set up Laravel MustVerifyEmail Contract In Your Model
Now, you need to implements MustVerifyEmail in User Model. Open the App/Models/User.php file and update as follows:
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable implements MustVerifyEmail
{
use HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
Step 3: Set the Email Verification Routes
Next,you need to add routes in app/routes/web.php file.
Auth::routes(['verify' => true]);
Step 4: Set the Email Verification Middleware
you can add verified Middleware HomeController or any controller which you want to not access any without verified user you can inject _construct method.
public function __construct()
{
$this->middleware(['auth', 'verified']);
}
Personally i Prefer to add verified in routes in app/routes/web.php file. using group Middlweware.
Route::group(['middleware' => ['auth', 'verified']], function () {
Route::get('/home', [App\Http\Controllers\HomeController::class, 'index'])->name('home');
});
After Registration you got this message.
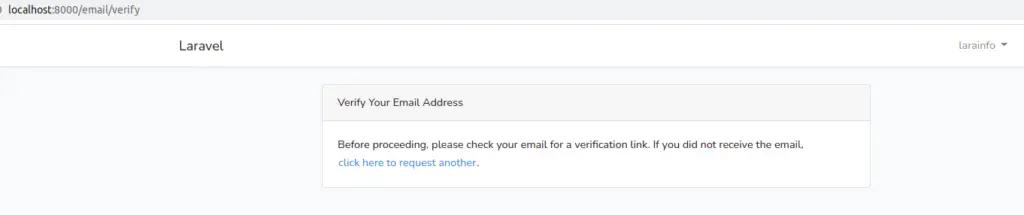
Next,you need to click Verify Email Address.
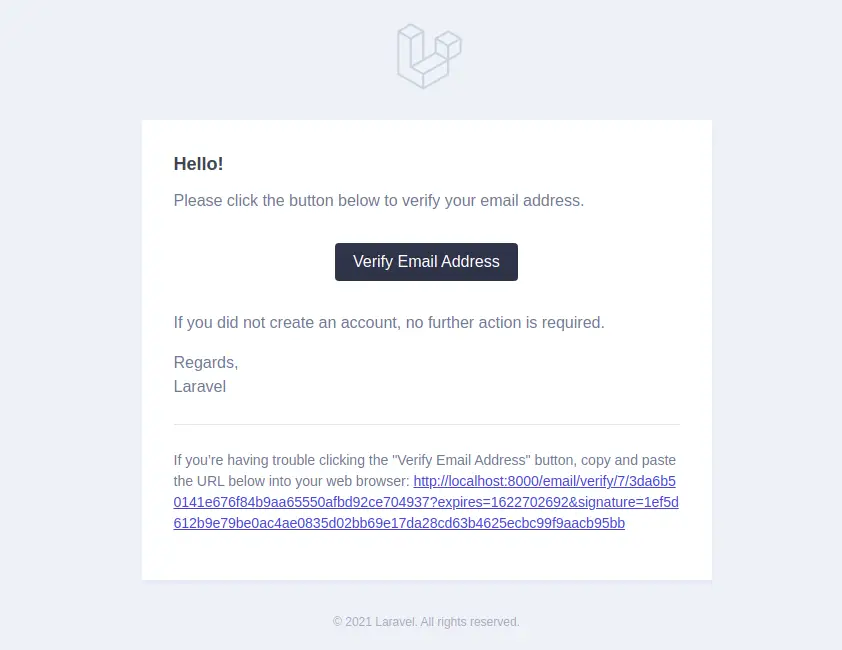
Now you can access the home and other pages.

[…] Laravel Email Verification with Laravel UI […]