In this tutorial, you will see how to implement email verification with Laravel Breeze. Laravel Breeze is a simple and minimal authentication scaffolding. It comes with default Blade components, Tailwind CSS, and Alpine.js.
Step 1: Set up Configuring the SMTP Server
You need to add the SMTP credentials in the .env file. In this section, I use Mailtrap, but you can use any mail service. Simply register for a Mailtrap account, go to the index page, find Integrations, select the Laravel section, and add these credentials to your .env file.
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=<YOUR_MAILTRAP_USERNAME>
MAIL_PASSWORD=<YOUR_MAILTRAP_PASSWORD>
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=<YOUR_Email_Address>
MAIL_FROM_NAME="${APP_NAME}"
Step 2: Set up Laravel MustVerifyEmail Contract In Your Model
Now, you need to implement MustVerifyEmail
in the User model. Open the App/Models/User.php
file and update it as follows:
<?php
namespace App\Models;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable implements MustVerifyEmail
{
use HasFactory, Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name',
'email',
'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password',
'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
Step 3: Set the Email Verification Middleware
Next, you need the just add verified middleware
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth','verified'])->name('dashboard');
require __DIR__.'/auth.php';
After this, you can check if a new user is registered.
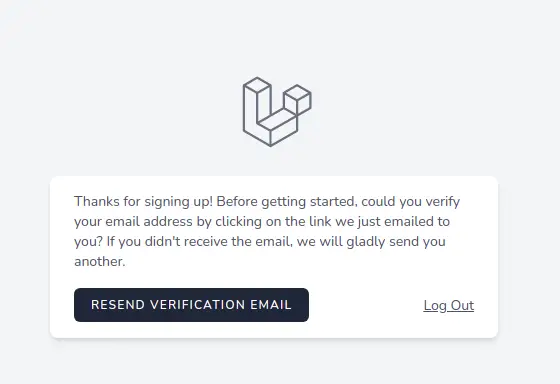
Then you email message.
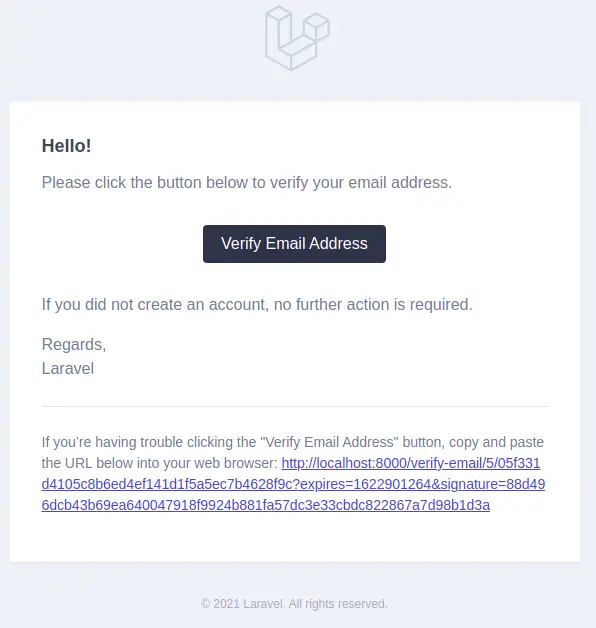