In this tutorial, we will learn how to upload an image in a REST API using Laravel 10. We will also validate the image before uploading it.
Step 1: Create Laravel Project
Run below command to create laravel project.
composer create-project laravel/laravel laravel-wishlist
Now, you need to connect the Laravel app to the database. Open the .env configuration file and add the database credentials as suggested below.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_user_name
DB_PASSWORD=database_password
Step 2: Create Image Model and Migration
Run shorthand command to create image modal, migration and controller.
php artisan make:model Image -m
create_images_table.php
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('images', function (Blueprint $table) {
$table->id();
$table->string('image');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('images');
}
};
app/Models/Image.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Image extends Model
{
use HasFactory;
protected $fillable = [
'image'
];
}
Step 3: Create Image Api Controller and Route
There are two ways to store and validate image files:
1. First Method: Image File Upload with Validation.
Create an Image API Controller by running the following command:
php artisan make:controller Api/ImageController
First you need to import Image class and import use Symfony\Component\HttpFoundation\Response
;
app/Http/Controllers/Api/ImageController.php
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Models\Image;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
class ImageController extends Controller
{
public function imageStore(Request $request)
{
$this->validate($request, [
'image' => 'required|image|mimes:jpg,png,jpeg,gif,svg|max:2048',
]);
$image_path = $request->file('image')->store('image', 'public');
$data = Image::create([
'image' => $image_path,
]);
return response($data, Response::HTTP_CREATED);
}
}
2. Second Way to Image file upload with validation.
Create image store request for validation.
php artisan make:request ImageStoreRequest
app/Http/Controllers/Requests/ImageStoreRequest.php
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class ImageStoreRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'image' => 'required|image|mimes:jpg,png,jpeg,gif,svg|max:2048',
];
}
}
Now you need remove Request and import ImageStoreRequest
for image validation.
app/Http/Controllers/Api/ImageController.php
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use App\Http\Requests\ImageStoreRequest;
use App\Models\Image;
use Illuminate\Http\Request;
use Symfony\Component\HttpFoundation\Response;
class ImageController extends Controller
{
public function imageStore(ImageStoreRequest $request)
{
$validatedData = $request->validated();
$validatedData['image'] = $request->file('image')->store('image');
$data = Image::create($validatedData);
return response($data, Response::HTTP_CREATED);
}
}
Now you need to set api.php
route.
routes/api.php
<?php
use App\Http\Controllers\Api\ImageController;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
Route::post('image',[ImageController::class, 'imageStore']);
Step 4: Migrate database & Storage links
run migrate database.
php artisan migrate
Storage links to public directory.
php artisan storage:link
Step 5: Setup and Test Api
First, navigate to the body section and select the form field for API image upload. Then, make a POST request to http://localhost:8000/api/image
and set the header Accept
to application/json
.
http://localhost:8000/api/image

Laravel 10 rest api Image upload validation test.
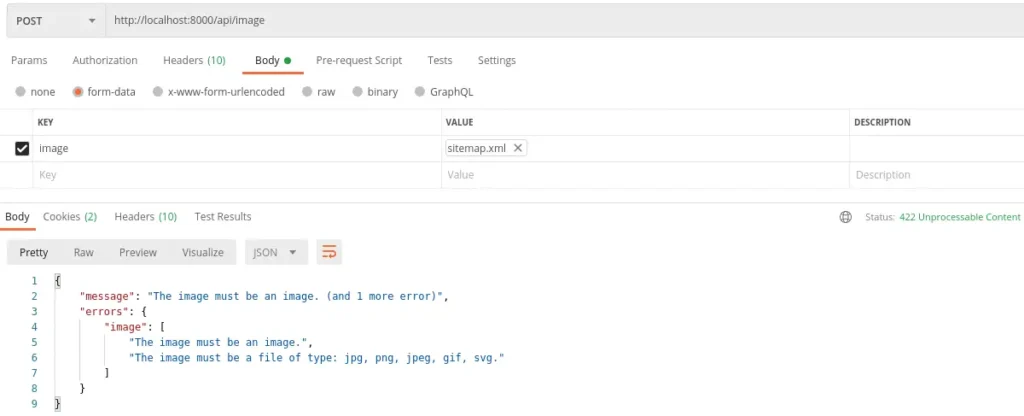
laravel 10 rest api Image upload successful.
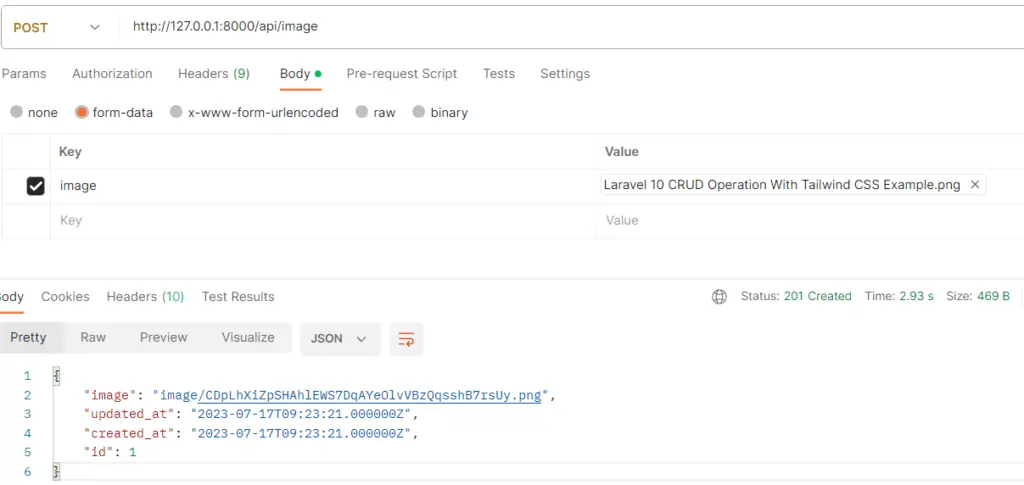