In this section we will install step by step vue 3 with bootstrap 5.
Setup Vue 3 Project
Create Vue 3 Project.
vue create bootstrap-vue
Then you need to choose vuejs 3 below option.
Vue CLI v4.5.15
? Please pick a preset:
❯ s ([Vue 3] babel, router, eslint)
a ([Vue 3] babel, typescript, router, vuex, eslint)
Default ([Vue 2] babel, eslint)
Default (Vue 3) ([Vue 3] babel, eslint)
Manually select features
Next, move to project directory and run server.
cd bootstrap-vue
npm run serve
Install bootstrap 5 in vue 3
Run below command to install bootstrap 5.
npm install bootstrap
install popperjs
npm i @popperjs/core
Import bootstrap 5 in main.js
Next, you need to import bootstrap css and js in main.js for global use.
import "bootstrap/dist/css/bootstrap.min.css"
import { createApp } from "vue";
import App from "./App.vue";
import router from "./router";
createApp(App).use(router).mount("#app");
import "bootstrap/dist/js/bootstrap.js"
You can edit HelloWorld.vue or you can leave as it.
<template>
<div class="hello">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
name: "HelloWorld",
props: {
msg: String,
},
};
</script>
Next, put bootstrap 5 button class and check it working.
<template>
<div class="home">
<img alt="Vue logo" src="../assets/logo.png" />
<HelloWorld msg="Install Vue 3 with Bootstrap 5" />
<button type="button" class="btn btn-primary">Primary</button>
<button type="button" class="btn btn-secondary">Secondary</button>
<button type="button" class="btn btn-success">Success</button>
<button type="button" class="btn btn-danger">Danger</button>
<button type="button" class="btn btn-warning">Warning</button>
<button type="button" class="btn btn-info">Info</button>
<button type="button" class="btn btn-light">Light</button>
<button type="button" class="btn btn-dark">Dark</button>
<button type="button" class="btn btn-link">Link</button>
</div>
</template>
<script>
// @ is an alias to /src
import HelloWorld from '@/components/HelloWorld.vue';
export default {
name: 'Home',
components: {
HelloWorld,
},
};
</script>
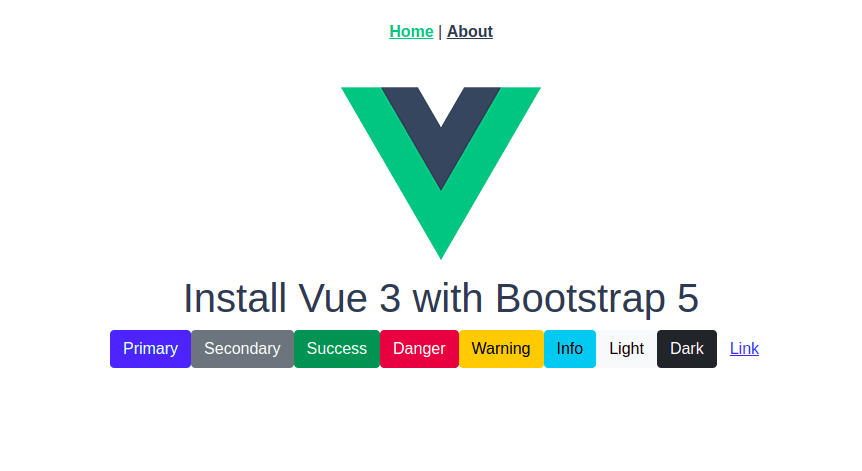
npm run serve