In modern web applications, smooth and responsive user interactions are crucial. When it comes to deleting records, we want to provide a seamless experience without full page reloads. Let’s explore how to implement this using Laravel 11, Inertia, and React.
Setting Up the Backend
First, let’s set up our Laravel backend to handle delete requests.
1. Define the Route
In your routes/web.php
file, add a delete route:
web.php
use App\Http\Controllers\ItemController;
Route::delete('/items/{item}', [ItemController::class, 'destroy'])->name('items.destroy');
2. Create the Controller Method
In your ItemController
, implement the destroy
method:
PHP
public function destroy(Item $item)
{
$item->delete();
return redirect()->route('items.index')->with('message', 'Item deleted successfully');
}
This method deletes the item and redirects back to the index page with a success message.
Implementing the Frontend
Now, let’s create a React component that will handle the delete functionality on the client side.
1. Create the React Component
import React from 'react';
import { router } from '@inertiajs/react';
export default function ItemList({ items }) {
const handleDelete = (id) => {
if (confirm('Are you sure you want to delete this item?')) {
router.delete(`/items/${id}`, {
preserveState: true,
preserveScroll: true,
});
}
};
return (
<div>
<h1>Items</h1>
<ul>
{items.map((item) => (
<li key={item.id}>
{item.name}
<button onClick={() => handleDelete(item.id)}>Delete</button>
</li>
))}
</ul>
</div>
);
}
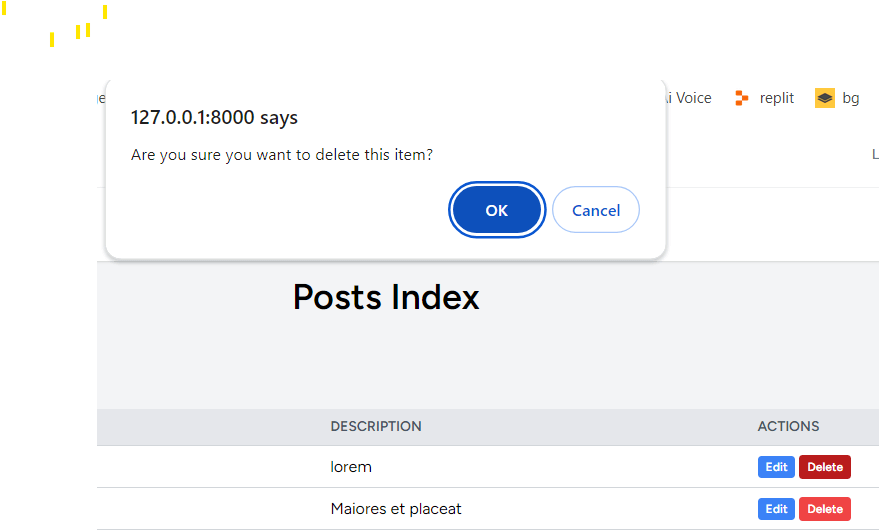
2. Understanding the Code
Let’s break down the key parts of this component:
- We import
router
from@inertiajs/react
, which allows us to make Inertia requests. - The
handleDelete
function is called when the delete button is clicked. It first confirms the action with the user. - If confirmed, we use
router.delete()
to send a DELETE request to our backend. - The
preserveState
andpreserveScroll
options maintain the current page state and scroll position after the delete operation.