In this tutorial, we will see how to use background images in React.js with Tailwind CSS. We’ll cover various examples of background images with Tailwind CSS & React.
Tool Use
React JS
Tailwind CSS
Example 1
React with tailwind css background image using inline style.
export default function Images() {
return (
<>
<div className="flex justify-center items-center h-screen">
<div
className="bg-cover bg-center h-80 w-96"
style={{
backgroundImage:
"url('https://cdn.pixabay.com/photo/2023/01/23/09/26/cat-7738210__340.jpg')",
}}
></div>
</div>
</>
);
}
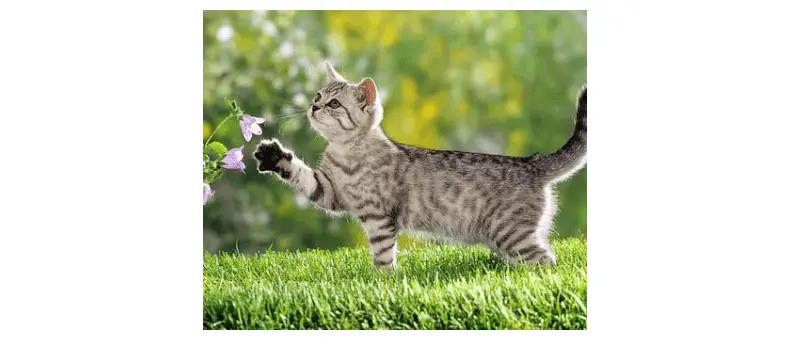
You can also define an image variable, which makes it much easier to read and understand the code.
export default function Images() {
const imageURL = "https://cdn.pixabay.com/photo/2023/01/23/09/26/cat-7738210__340.jpg";
return (
<>
<div className="flex justify-center items-center h-screen">
<div
className="bg-cover bg-center h-80 w-96"
style={{
backgroundImage: `url(${imageURL})`,
}}
></div>
</div>
</>
);
}
Example 2
You can use a background image in React by utilizing Tailwind’s bg-url
class.
export default function Images() {
return (
<>
<div className="flex justify-center items-center h-screen">
<div className='bg-[url("https://cdn.pixabay.com/photo/2023/01/23/09/26/cat-7738210__340.jpg")] bg-cover bg-center h-80 w-96'></div>
</div>
</>
);
}